Note
Click here to download the full example code
Cross-session motor imagery with deep learning EEGNet v4 model#
This example shows how to use BrainDecode in combination with MOABB evaluation. In this example, we use the architecture EEGNetv4.
# Authors: Igor Carrara <igor.carrara@inria.fr>
# Bruno Aristimunha <b.aristimunha@gmail.com>
#
# License: BSD (3-clause)
import matplotlib.pyplot as plt
import mne
import seaborn as sns
import torch
from braindecode import EEGClassifier
from braindecode.models import EEGNetv4
from sklearn.pipeline import make_pipeline
from skorch.callbacks import EarlyStopping, EpochScoring
from skorch.dataset import ValidSplit
from moabb.datasets import BNCI2014_001
from moabb.evaluations import CrossSessionEvaluation
from moabb.paradigms import MotorImagery
from moabb.utils import setup_seed
mne.set_log_level(False)
# Print Information PyTorch
print(f"Torch Version: {torch.__version__}")
# Set up GPU if it is there
cuda = torch.cuda.is_available()
device = "cuda" if cuda else "cpu"
print("GPU is", "AVAILABLE" if cuda else "NOT AVAILABLE")
Torch Version: 1.13.1+cu117
GPU is NOT AVAILABLE
In this example, we will use only the dataset BNCI2014_001
.
Running the benchmark#
This example uses the CrossSession evaluation procedure. We focus on the dataset BNCI2014_001 and only on 1 subject to reduce computational time.
To keep the computational time low, the epoch is reduced. In a real situation, we suggest using the following: EPOCH = 1000 PATIENCE = 300
This code is implemented to run on the CPU. If you’re using a GPU, do not use multithreading (i.e. set n_jobs=1)
# Set random seed to be able to reproduce results
seed = 42
setup_seed(seed)
# Ensure that all operations are deterministic on GPU (if used) for reproducibility
torch.backends.cudnn.deterministic = True
torch.backends.cudnn.benchmark = False
# Hyperparameter
LEARNING_RATE = 0.0625 * 0.01 # parameter taken from Braindecode
WEIGHT_DECAY = 0 # parameter taken from Braindecode
BATCH_SIZE = 64 # parameter taken from BrainDecode
EPOCH = 10
PATIENCE = 3
fmin = 4
fmax = 100
tmin = 0
tmax = None
# Load the dataset
dataset = BNCI2014_001()
events = ["right_hand", "left_hand"]
paradigm = MotorImagery(
events=events, n_classes=len(events), fmin=fmin, fmax=fmax, tmin=tmin, tmax=tmax
)
subjects = [1]
X, _, _ = paradigm.get_data(dataset=dataset, subjects=subjects)
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
Create Pipelines#
In order to create a pipeline, we need to load a model from braindecode.
the second step is to define a skorch model using EEGClassifier from braindecode
that allows converting the PyTorch model in a scikit-learn classifier.
Here, we will use the EEGNet v4 model 1 .
This model has mandatory hyperparameters (the number of channels, the number of classes,
and the temporal length of the input) but we do not need to specify them because they will
be set dynamically by EEGClassifier using the input data during the call to the .fit()
method.
# Define a Skorch classifier
clf = EEGClassifier(
module=EEGNetv4,
optimizer=torch.optim.Adam,
optimizer__lr=LEARNING_RATE,
batch_size=BATCH_SIZE,
max_epochs=EPOCH,
train_split=ValidSplit(0.2, random_state=seed),
device=device,
callbacks=[
EarlyStopping(monitor="valid_loss", patience=PATIENCE),
EpochScoring(
scoring="accuracy", on_train=True, name="train_acc", lower_is_better=False
),
EpochScoring(
scoring="accuracy", on_train=False, name="valid_acc", lower_is_better=False
),
],
verbose=1, # Not printing the results for each epoch
)
# Create the pipelines
pipes = {}
pipes["EEGNetV4"] = make_pipeline(clf)
Evaluation#
dataset.subject_list = dataset.subject_list[:2]
evaluation = CrossSessionEvaluation(
paradigm=paradigm,
datasets=dataset,
suffix="braindecode_example",
overwrite=True,
return_epochs=True,
n_jobs=1,
)
results = evaluation.process(pipes)
print(results.head())
BNCI2014-001-CrossSession: 0%| | 0/2 [00:00<?, ?it/s]/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
epoch train_acc train_loss valid_acc valid_loss dur
------- ----------- ------------ ----------- ------------ ------
1 0.5156 0.7659 0.5172 0.6932 0.2677
2 0.5781 0.7363 0.5172 0.6925 0.1820
3 0.4375 0.7591 0.5517 0.6919 0.1816
4 0.5938 0.6669 0.5172 0.6913 0.1830
5 0.5781 0.6621 0.5172 0.6907 0.1825
6 0.5312 0.7085 0.4828 0.6903 0.2363
7 0.5469 0.7184 0.5172 0.6900 0.1833
8 0.4531 0.7167 0.5172 0.6897 0.1832
9 0.5938 0.6647 0.5172 0.6894 0.1820
10 0.5156 0.6890 0.5172 0.6890 0.1820
epoch train_acc train_loss valid_acc valid_loss dur
------- ----------- ------------ ----------- ------------ ------
1 0.5156 0.7365 0.4138 0.7091 0.1892
2 0.5781 0.6883 0.4483 0.7075 0.1832
3 0.6094 0.6824 0.4483 0.7060 0.1830
4 0.5312 0.7151 0.4138 0.7049 0.1823
5 0.4844 0.7221 0.4138 0.7040 0.1828
6 0.5469 0.7016 0.4483 0.7032 0.2385
7 0.5781 0.6593 0.4483 0.7025 0.1846
8 0.5469 0.6934 0.4828 0.7019 0.1837
9 0.5469 0.6745 0.4828 0.7014 0.1833
10 0.5469 0.6874 0.4828 0.7010 0.1811
BNCI2014-001-CrossSession: 50%|##### | 1/2 [00:06<00:06, 6.90s/it]/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
/home/runner/work/moabb/moabb/moabb/datasets/preprocessing.py:279: UserWarning: warnEpochs <Epochs | 24 events (all good), 2 – 6 s (baseline off), ~4.1 MB, data loaded,
'right_hand': 12
'left_hand': 12>
warn(f"warnEpochs {epochs}")
epoch train_acc train_loss valid_acc valid_loss dur
------- ----------- ------------ ----------- ------------ ------
1 0.4219 0.7449 0.6207 0.6832 0.1812
2 0.4688 0.7881 0.6207 0.6865 0.1797
3 0.5000 0.7344 0.6207 0.6874 0.1762
Stopping since valid_loss has not improved in the last 3 epochs.
epoch train_acc train_loss valid_acc valid_loss dur
------- ----------- ------------ ----------- ------------ ------
1 0.4844 0.7113 0.5517 0.6901 0.2399
2 0.4531 0.7591 0.5517 0.6905 0.1755
3 0.4531 0.7438 0.5517 0.6908 0.1781
Stopping since valid_loss has not improved in the last 3 epochs.
BNCI2014-001-CrossSession: 100%|##########| 2/2 [00:11<00:00, 5.36s/it]
BNCI2014-001-CrossSession: 100%|##########| 2/2 [00:11<00:00, 5.59s/it]
score time samples ... n_sessions dataset pipeline
0 0.521026 2.200796 144.0 ... 2 BNCI2014-001 EEGNetV4
1 0.494985 2.145613 144.0 ... 2 BNCI2014-001 EEGNetV4
2 0.493441 0.897470 144.0 ... 2 BNCI2014-001 EEGNetV4
3 0.544367 0.953355 144.0 ... 2 BNCI2014-001 EEGNetV4
[4 rows x 9 columns]
Plot Results#
plt.figure()
sns.barplot(data=results, y="score", x="subject", palette="viridis")
plt.show()
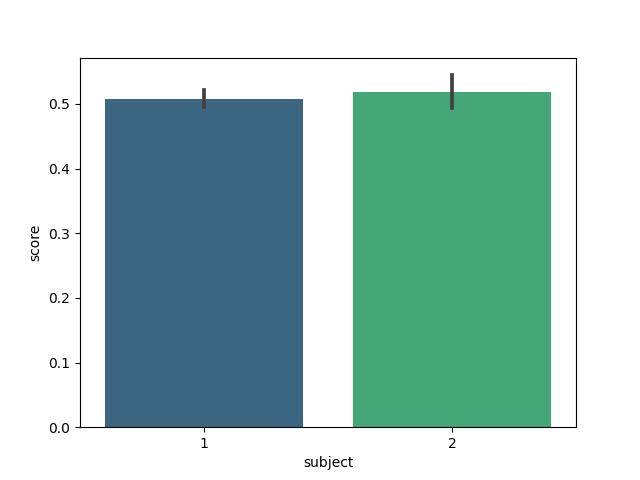
References#
- 1
Lawhern, V. J., Solon, A. J., Waytowich, N. R., Gordon, S. M., Hung, C. P., & Lance, B. J. (2018). EEGNet: a compact convolutional neural network for EEG-based brain-computer interfaces. Journal of neural engineering, 15(5), 056013.
Total running time of the script: ( 0 minutes 18.269 seconds)
Estimated memory usage: 277 MB